Demo
var
otslider =
new
OTSlider();
otslider.init();
Getting Started
otSlider is written with vanilla javascript also know as plain or pure javascript. Which means that you don't need an external library to run it.
How To
In order to run otSlider, it's easy. Just download it, then uncompress/extract the files inside the downloaded file archive and upload it to your Web Application.
After upload otSlider's files into your Web Application, do the following steps:
Step 1:
Call otslider.css and otslider.js files at the <head> or <body> tag.
<>
<>
...
< rel="stylesheet" type="text/css" href="https://yoursite.com/otslider/otslider.css">
< src="https://yoursite.com/otslider/otslider.js">
...
</>
<>
...
</>
</>
Step 2:
Now that you've called the needed otSlider files within <head> or <body> tag. It's time to initialize it.
otSlider can be initialized within <head> or <body> tag.
<>
<>
...
< rel="stylesheet" type="text/css" href="https://yoursite.com/otslider/otslider.css">
< src="https://yoursite.com/otslider/otslider.js">
...
</>
<>
...
<>
var
otslider
= new
OTSlider();
otslider.init();
</>
...
</>
</>
Optional configs object
otSlider can be initialized with an optional object as argument, which is used to alter some functions of the slider.
Note: The optional configs object is only called on init() method.
Bellow are the default configs of the object:
var otslider = new OTSlider();
otslider.init({
element : 'ot-slider',
direction : 'ltr',
transition : 'slide',
transitionTiming : 'ease',
prevButton : '«',
nextButton : '»',
duration : 2000,
transitionDuration : 500,
autoPlay : true,
pauseOnHover : true,
showPrevNext : true,
showNav : true,
swipe : true,
responsive : true,
roundButtons : false,
numericNav : true
itemsToShow : 1
itemsScrollBy : 1
padding : 0
teasing : 0
swipeFreely : false
centered : false
});
Where:
Imp. Version | Object Key | Type | Expected Value | Description |
---|---|---|---|---|
initial | element | object | string | It can be an HTML elemnt or its class name or its ID. | |
initial | direction | string | ltr | rtl | Indicate the direction that otSlider must perform. |
initial | transition | string | slide | fade | Indicate the type of transition that otSlider must use. |
initial | prevButton | string | A string that will be displayed on previous button. | |
initial | nextButton | string | A string that will be displayed on next button. | |
initial | duration | number | integer | The amount of time in milliseconds, that each item is displayed. |
initial | transitionDuration | number | integer | The amount of time in milliseconds, that the transition takes. |
v1.1.0 | transitionTiming | string | ease | ease-out | ease-in | linear | cubic-bezier(...) | Determine how the the transition must behaviour. This will only take effect on all modern Web Browsers that support CSS3 transition property. |
initial | autoPlay | boolean | true | false | If set to true (default), OTSlider will automatically plays. Else will only play if one of its buttons get clicked. |
initial | pauseOnHover | boolean | true | false | It tells OTSlider to suspend autoPlay while the user put the mouse pointer or finger above it. |
initial | showPrevNext | boolean | true | false | It tells OTSlider to show or hide the previous and next buttons. |
initial | showNav | boolean | true | false | It tells OTSlider to show or hide the navigation list of all items within it. |
initial | swipe | boolean | true | false | It enable or disable swipe feature. |
initial | responsive | boolean | true | false | Tells OTSlider to adapt its width or height to diferent devices screens. |
v1.1.0 | roundButtons | boolean | true | false | If set to true, all the buttons within the slider will be round. Else will be rectangular or square. |
v1.1.0 | numericNav | boolean | true | false | If set to true, the nav will be numbered according the number of items. |
initial | width | number | integer | float | Set OTSlider's custom width |
initial | height | number | integer | float | Set OTSlider's custom height |
v2.0.0 | *slide itemsToShow | number | integer | Determine the total number of items that must be displayed at once. |
v2.0.0 | *slide itemsScrollBy | number | integer | Determine by how many items otSlider must scroll. Note that the value must be greeter than 0 and less or iquals to the "itemsToShow". |
v2.0.0 | *slide padding | number | integer | The space in pixels between slider's items. |
v2.0.0 | *slide teasing | number | integer | The portion in pixels of the previous or next items to be displayed. |
v2.0.0 | *slide swipeFreely | boolean | true | false | If set to true the slider will not fallback after swipe. It will stay at the last swipe position. |
v2.0.0 | *slide centered | boolean | true | false | If set to true the current displayed items (determined by itemsToShow) will be centralised. |
(*slide) symbol: Indicate the features that will only take effect if otSlider is set to use "slide" transition.
More demos
Bellow are some demos of OTSlider initialized with optional object parameter.
Fade Transition:
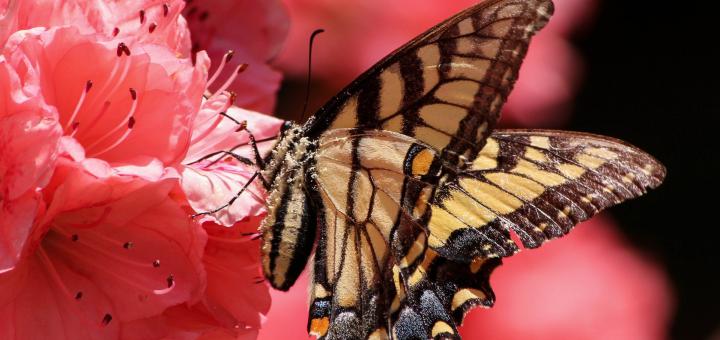
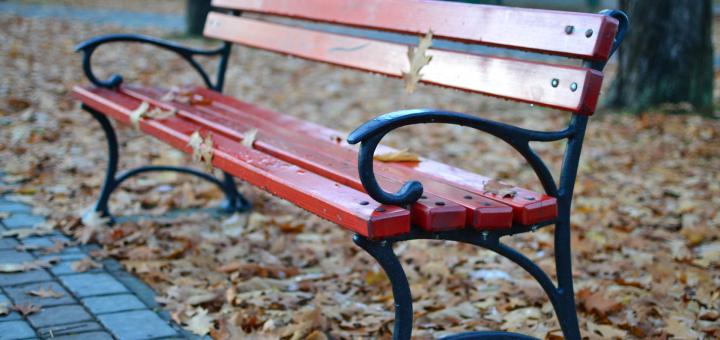
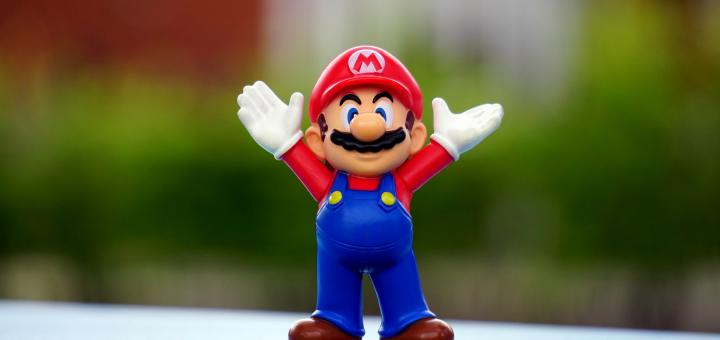
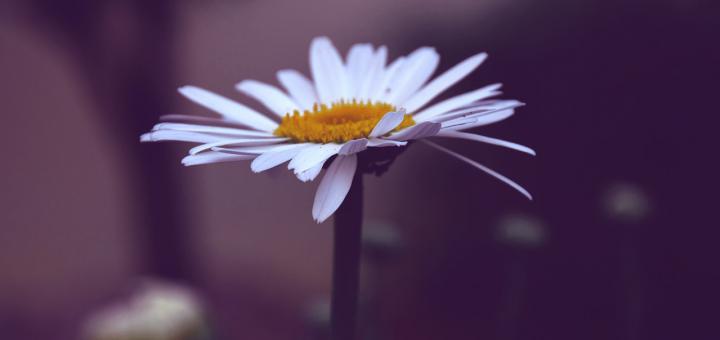
var otslider = new OTSlider();
otslider.init({
element : document.getElementById('fade-slider'),
transition : 'fade'
});
Right To Left (rtl) direction and Round Buttons:
var otslider = new OTSlider();
otslider.init({
element : 'rb-slider',
direction : 'rtl',
duration : 4500,
transitionDuration : 1500,
roundButtons : true,
numericNav : false
});
Disable autoPlay and hide nav (navigation):
var otslider = new OTSlider();
otslider.init({
element : 'nap-slider',
autoPlay : false,
roundButtons : true,
showNav : false
});
Show a portion of next item and also give some space between items:
var otslider = new OTSlider();
otslider.init({
element : "tp-slider",
teasing : 60,
padding : 4,
roundButtons : true
});
Show a portion of previous or next items and give some space between items:
var otslider = new OTSlider();
otslider.init({
element : "centered-slider",
teasing : 75,
padding : 6,
transitionDuration : 1000,
centered : true
roundButtons : true
});
Show multiple items at once and multiple scroll:
var otslider = new OTSlider();
otslider.init({
element : "mi-slider",
itemsToShow : 3,
itemsScrollBy : 3,
teasing : 120
padding : 6
duration : 5000
centered : true
roundButtons : true
numericNav : false
});
Show multiple items at once and Swipe Freely:
var otslider = new OTSlider();
otslider.init({
element : "sf-slider",
itemsToShow : 4,
teasing : 60
padding : 6
swipeFreely : true
autoPlay : false
showNav : false
showPrevNext : false
});